PROJECTS
The project examples in this section show and demonstrate how easy and feasible is the Generative AI adoption in any process. Every process can consume Generative AI Service by invoking REST API services exposed by a trusted Service Provider.
The codes on this site, contains functions already published in the literature, and are not intended to be uses as-is. If you like adopt them, is your responsibility to check whatever needed in your environment and modify / integrate them accordingly to the requirements of your organization to build functions that are compliant with your company policies and country laws.
Invoking IBM watsonx API
This is a sample code to invoke Generative AI services via REST API powered by IBM watsonx. It then shows the Payload returned as the result of REST API request submitted. The integration and the execution of a REST API code to invoke Generative AI services based on the Large Language Model available in watsonx is straight and easy to implement. The code submits a request for generating a recipe for vegan banana bread, then it displays the output in row format to show also the metadata that the API returns to the consumer.
The code and the comments are in original language.
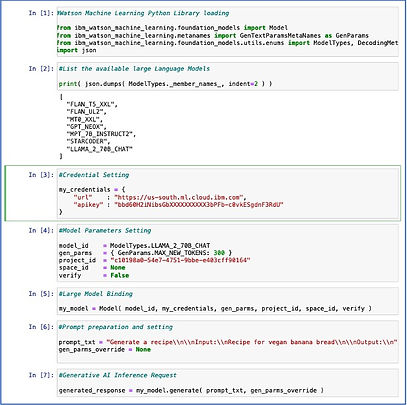
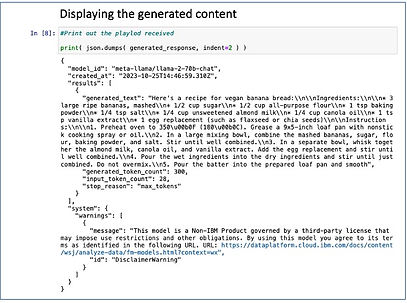
Python Code
#Watson Machine Learning Python Library loading
from ibm_watson_machine_learning.foundation_models import Model
from ibm_watson_machine_learning.metanames import GenTextParamsMetaNames as GenParams
from ibm_watson_machine_learning.foundation_models.utils.enums import ModelTypes, DecodingMethods
import json
#List the available large Language Models
print( json.dumps( ModelTypes._member_names_, indent=2 ) )
#Credential Setting
my_credentials = {
"url" : "https://us-south.ml.cloud.ibm.com",
"apikey" : "Insert_Here_Your_Key"
}
#Model Parameters Setting
model_id = ModelTypes.LLAMA_2_70B_CHAT
gen_parms = { GenParams.MAX_NEW_TOKENS: 300 }
project_id = "c10198a0-54e7-4751-9bbe-e403cff90164"
space_id = None
verify = False
#Large Model Binding
my_model = Model( model_id, my_credentials, gen_parms, project_id, space_id, verify )
#Prompt preparation and setting
prompt_txt = "Generate a recipe\\n\\nInput:\\nRecipe for vegan banana bread\\n\\nOutput:\\n"
gen_parms_override = None
#Generative AI Inference Request
generated_response = my_model.generate( prompt_txt, gen_parms_override )
## Displaying the generated content
#Print out the paylod received
print( json.dumps( generated_response, indent=2 ) )
Invoking OpenAI API
This is a sample code to invoke Generative AI services via REST API powered by OpenAI. It also shows the Payload returned as the result of REST API request submitted. The integration and the execution of a REST API code to invoke Generative AI services based on the OpenAI Large Language Model.
The code submits a request for generating a response on what is the best building technique against headquarters. It than displays the output the API returns to the consumer.
The code and the comments are in original language.
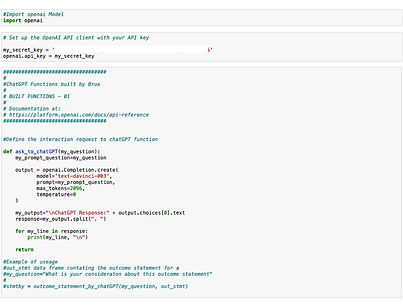

Python Code
#Import openai Model
import openai
# Set up the OpenAI API client with your API key
my_secret_key = ‘xxxxxxxxxxxxxxxxxxxxxxxxxx’
openai. api_key = my_secret_key
#######################
#
#ChatGPT Functions built by Brux
#
# BUILT FUNCTIONS - 01|
#
# Documentation at:
# https://platform.openai.com/docs/api-reference
########################
#Define the interaction request to chatGPT function
def ask_to_chatGPT(my_question) :
my_prompt_question=my_question
output = openai.Completion.create(
model=' text-davinci-003',
prompt=my_prompt_question,
max_tokens=2096,
temperature=0
)
my_output="\nChatGPT Response:" + output.choices [0]. text
response=my_output.split(". “)
for my_line in response:
print (my_line, "\n")
return
#Example of useage
#out_stmt data frame contating the outcome statement for a
#my_question="What is yuur consideraton about this outcome statement"
#
#stmtby = outcome_statement_by_chatGPT(my_question, out_stmt)
my_question ="what is the best building technique against headquarters?"
ask_to_chatGPT (my_question)